Python- Numpy Starter
Code effortlessly.

Numpy stands for Numerical Python. It is the library most suited if one wishes to work with matrices, arrays, transformations etc. Its simplicity and highly efficient algorithms have made numpy a must learn library.
Let us conduct an experiment to see how numpy performs over pythons ‘for loop’ :
In the below experiment we are trying to add corresponding elements in two arrays, i.e. 1st element of one array to 1st element of second array and likewise. At the end we can compare the time taken to complete the task.
from datetime import datetime
a = [2,3,4]*100000000
b = [2,4,4]*100000000
start = datetime.now()
c = []
for ai,bi in zip(a,b):
c.append(ai+bi)#total time taken
print(datetime.now()-start)

Using numpy to solve the same problem statement.
from datetime import datetime
import numpy as np
a = [2,3,4]*100000000
b = [2,4,4]*100000000
start = datetime.now()
a = np.array(a)
b = np.array(b)
c = a+b#total time taken
print(datetime.now()-start)
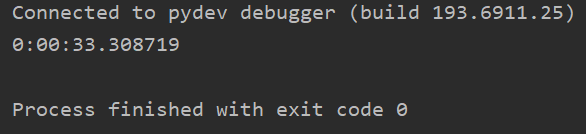
We can conclude that numpy outperforms ‘for loops’ with very high margin.
Arrays:
“At the core of the NumPy package, is the ndarray object. This encapsulates n-dimensional arrays of homogeneous data types, with many operations being performed in compiled code for performance.” — Numpy documentation.
import numpy as np
a = np.array([1, 2, 3])
print("Type of the object: {} and shape: {}".format(type(a),a.shape))
print("elements in array {} {} {}".format(a[0], a[1], a[2]))
'''Changing values of element in the array'''
a[0] = 5
print("altered array: {}".format(a))
'''2D array'''
b = np.array([[1,2,3],[4,5,6]])
print("shape of the 2d array: {} ".format(b.shape))
print("{}, {}, {}".format(b[0, 0], b[0, 1], b[1, 0]))

We can create different type of numpy arrays by calling simple numpy methods.
import numpy as np
# creating matrix from numpy methods
ones = np.ones((2,2))
print(ones)
zeros = np.zeros((2,3))
print(zeros)
mat = np.full((2,1), 7)
print(mat)
r_mat = np.random.random((3,3)) #creating random matrix
print(r_mat)
I = np.eye(3) #identity matrix
print(I)

Numpy Indexing:
import numpy as np
np_matrix = np.random.randint(low=1,high=100,size=(5,5))
print(np_matrix)
# to get the first row
print(np_matrix[0,:])
# to get the first two rows
print(np_matrix[0:2,:])
# to get the first two rows and 2 columns
print(np_matrix[0:2,:2])
# to get first element
print(np_matrix[0,0])

Algebra of arrays:
import numpy as np
x = np.random.randint(low=1,high=10,size=(2,2))
y = np.random.randint(low=1,high=10,size=(2,2))
print(x + y)
print(np.add(x, y))
print(x - y)
print(np.subtract(x, y))
print(x * y)
print(np.multiply(x, y))
print(x / y)
print(np.divide(x, y))
print(np.sqrt(x))

Broadcasting of arrays:
import numpy as np
x = np.random.randint(low=1,high=10,size=(3,5))
print(x)
y = np.random.randint(low=1,high=10,size=(1,5))
print("\n",y)
print("\n",x+y)

The ‘x+y’ will work even if x has shape (3,5) and y has shape (1,5) due to broadcasting. Y will actually have a shape (3,5), where each row was a copy of y, and then the elementwise summation will be performed.
Stacking of arrays:
Stack functions are helpful when we want merge two n-dim arrays (vertically or horizontally).
import numpy as np
x = np.random.randint(low=1,high=10,size=(2,2))
y = np.random.randint(low=1,high=10,size=(2,2))
print(x)
print(y)
z = np.hstack((x,y))
print('\n',z)
v = np.vstack((x,y))
print('\n',v)

Vectorizing functions via numpy:
To speed up operations on elements of a matrix we can vectorize a python function and apply on the matrix.
“The vectorized function evaluates pyfunc over successive tuples of the input arrays like the python map function, except it uses the broadcasting rules of numpy.” — numpy documentation.
import numpy as np
def change_number(x):
return x**2 + 3
x = np.random.randint(low=1,high=10,size=(5,5))
print(x)
z = np.vectorize(change_number)
print("\n",z(x))

I hope the above concepts will help you code more efficiently using numpy arrays.
That’s all.